Qt is a framework for developing software with a GUI (and other software, too). It uses C++. QML (Qt Meta Language) is a declarative language for developing UI, and is based on JavaScript. I'll be using QML to build a prototype of a UI for a certain device. For that purpose, I'll be using Qt Creator that is installed on the Ubuntu 11.10. There are some new experiences here. I'll move on to connecting QML applications with C++ plugins later, but for now just some plain QML.
To create a simple QML project, I select File -> New File or Project from Qt Creator menu. From Projects I select Qt Quick Project and Qt Quick UI on the right.
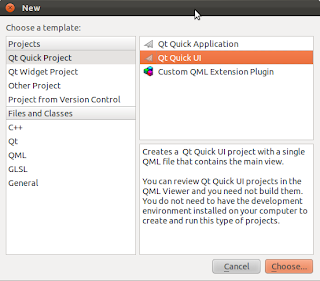
New Qt Creator Project
Next I select the location for the project files and when I'm done, the project contains only one qml file and a project file with the extension "qmlproject" which I do not need to know much at that early stage. The qml file contains a simple "Hello World" application.
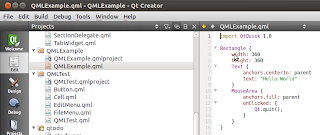
Qt Creator Environment
Compared to my experience, QML appears to be somewhat similar to WPF, considering that I define the layout with the declarative part and then can apply scripts additionally to provide extra functionality. In this first post, I'll only look at some of the most basic layout elements. I'm starting with the UI that has three panels: the left panel takes up one third of the space, and the right side is divided into the top and bottom parts, the bottom being one third of the vertical space. The screen size is hard-coded at this point.
The most basic element is a Rectangle. It is just that - a UI element of a rectangular shape. It can be filled with a color and can hold other UI elements. For example, it can hold a Text element which is, essentially, a label.
Rectangle{
id: listViewPanel
width: 100
height: 100
x: 0
y: 0
border.color: "black"
color: "green"
border.width: 1
Text{
text: "Left panel"
}
}
The id is optional, but will be used later to reference the element. Other parameters are self-explanatory.
Here is the first QML application - just a few rectangles that take up the screen.
import QtQuick 1.0
Rectangle{
id: main
color: "lightGrey"
property int rectWidth: 480
property int rectHeight: 480
width: rectWidth
height: rectHeight
Rectangle{
id: listViewPanel
width: rectWidth/3
height: rectHeight
x: 0
y: 0
border.color: "black"
color: "green"
border.width: 1
Text{
text: "Left panel"
}
}
Rectangle{
id: detailsPanel
width: parent.width*2/3
height: parent.height*2/3
x: parent.width/3
y: 0
border.color: "black"
color: "yellow"
border.width: 1
Text{
text: "Details panel"
}
}
Rectangle{
id: loggingPanel
width: parent.width*2/3
height: parent.height/3
border.color: "black"
border.width: 1
x: parent.width/3
y: parent.height*2/3
color: "blue"
Text{
text: "Logging panel"
}
}
}
Rectangle main is the parent element, and defines the size of the screen. It defines two properties, which are screen height and width and are used by child elements to calculate their own sizes. A child element can access the parent's properties by using parent.property syntax (i.e. parent.height). Each child rectangle has a Text element inside, just to provide some visual feedback.
Here's the result of executing the application:
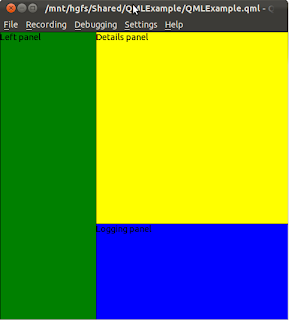
First QML Application
References:
Qt - Cross-platform application and UI frameworkQML Rectangle Element by Evgeny. Also posted on my website
No comments:
Post a Comment